The i18next-http-backend is a popular backend plugin for i18next, a widely used internationalization framework for JavaScript applications. It serves as a middleware to load translation files from a server over HTTP. However, developers often encounter an issue where the library makes multiple API calls, sometimes unnecessarily, leading to redundant network requests and potential performance degradation.
Understanding the Issue
The primary reason i18next-http-backend sends multiple API requests is due to incorrect configuration or unintended reload triggers. The common causes include:
- Missing or incorrect caching settings: If caching is not enabled or properly configured, the library may attempt to fetch the translations multiple times.
- Multiple instances of i18next: When applications initialize i18next multiple times within the same session, each instance may trigger separate API requests.
- Backend retries on failure: By default, i18next-http-backend tries to load a resource multiple times if it fails initially.
- Language detection causing multiple loads: If language detection is set improperly, i18next may attempt to load translations for multiple language variations.
- HMR (Hot Module Replacement) in development: In a React or Webpack-powered environment, hot module replacement can cause translation files to be reloaded frequently.
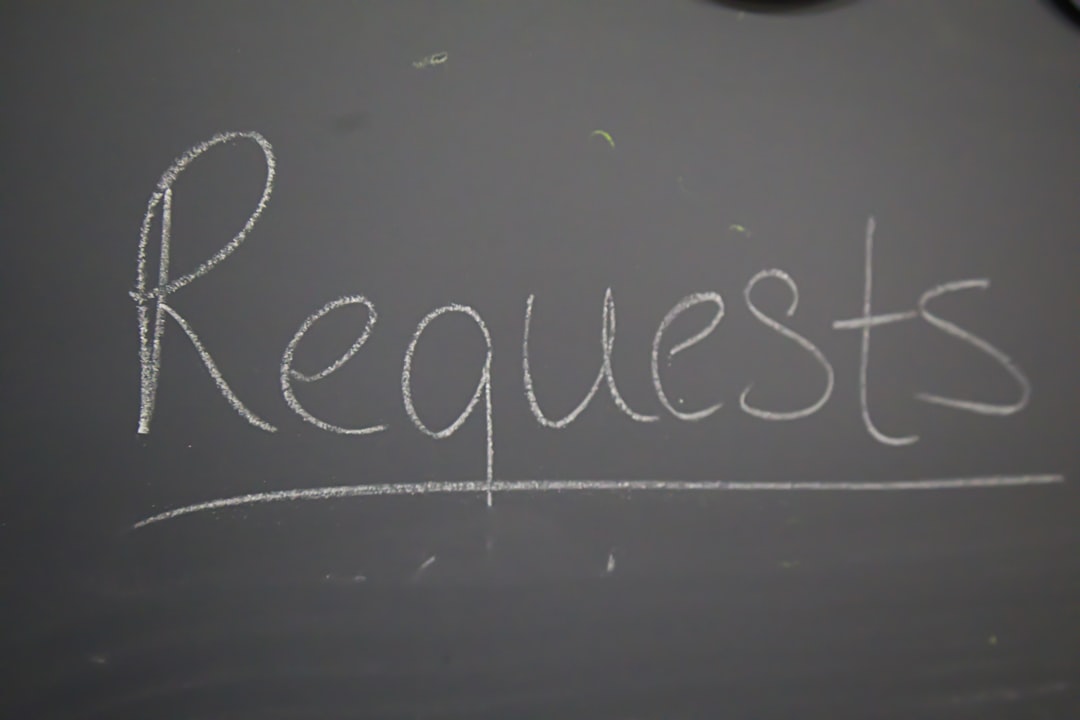
How to Fix the Multiple API Calls Issue
1. Enable Caching
Ensuring that translation files are cached can prevent redundant requests. The i18next library provides built-in caching mechanisms, but developers need to configure them correctly.
import i18n from 'i18next';
import HttpBackend from 'i18next-http-backend';
import { initReactI18next } from 'react-i18next';
i18n
.use(HttpBackend)
.use(initReactI18next)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
cache: true // Enable caching
},
debug: false,
interpolation: {
escapeValue: false
}
});
export default i18n;
If using a browser-based setup, ensure that the server is setting proper cache-control headers in its API response.
2. Avoid Double Initialization
Check your application to ensure that i18next is only initialized once. In React applications, unintentional double initialization can occur if the setup is not structured properly.
3. Adjust Backend Retry Mechanism
If multiple calls happen due to failed attempts, modify the retry mechanism under the backend configuration:
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
allowMultiLoading: false, // Disable multiple retry attempts
requestOptions: {
mode: 'cors',
credentials: 'same-origin',
cache: 'default'
}
}

4. Configure Language Loading Properly
If i18next loads multiple variants of a language (e.g., en-US
and en
), it can trigger additional requests. To mitigate this, configure whitelist
or fallbackLng
settings properly:
i18n.init({
fallbackLng: 'en',
load: 'languageOnly', // Prevents loading en-US when 'en' is enough
backend: {
loadPath: '/locales/{{lng}}.json'
}
});
5. Prevent Hot Module Replacement from Triggering Requests
For React-based applications, HMR (Hot Module Replacement) can cause unexpected reloading of translation files. Disabling HMR for i18n files or preventing reloading in development mode can help.
if (module.hot) {
module.hot.accept();
}
Additionally, developers can implement caching mechanisms specifically in development mode to reduce re-fetching.
Conclusion
Fixing multiple API requests caused by i18next-http-backend requires proper configuration and monitoring of caching, retries, initialization, and language detection settings. A well-optimized setup ensures efficient language handling, reducing unnecessary API calls and improving the overall performance of applications.
FAQ
- Why is i18next fetching translation files multiple times?
- It might be due to missing caching, multiple instances of i18next, failed retries, or improper language detection settings.
- How can I enable caching for i18next-http-backend?
- The backend can be configured to use caching by ensuring proper HTTP headers and setting the
cache
option in the configuration. - Can I force i18next to load translations only once?
- Yes, by correctly setting up caching, disabling multiple retries, and ensuring only one initialization of i18next in the application.
- Does language detection affect multiple API calls?
- Yes, if language detection is not correctly configured, i18next may attempt to load multiple language variants unnecessarily.
- How does Hot Module Replacement impact i18next?
- HMR can cause translation files to reload frequently during development. Preventing HMR for i18next files can reduce unnecessary calls.